Table Of Content
One of these hierarchies (often called the Abstraction) will get a reference to an object of the second hierarchy (Implementation). The abstraction will be able to delegate some (sometimes, most) of its calls to the implementations object. Since all implementations will have a common interface, they’d be interchangeable inside the abstraction. The Bridge pattern decouples an abstraction from its implementation, sothat the two can vary independently. A household switch controllinglights, ceiling fans, etc. is an example of the Bridge. The actual switch can beimplemented as a pull chain, simple two position switch, or a varietyof dimmer switches.
Mastering Design Patterns — 08: A Comprehensive Guide to the Bridge Pattern
Create a Question interface that provides the navigation from one question to another or vice-versa. To build a clean architecture in C#, laying a solid foundation for your codebase is important. One of the fundamentals of clean architecture in C# is adherence to the SOLID principles, which serve as... It allows users to register and write a few things about their daily routine and interactions with their close friends, just like a daily journal. This software will require no user authentication or instantiation, and you would consider that the user will be entered immediately into the system for simplicity.
Understanding the Problem
The Device classes act as the implementation, whereas the Remotes act as the abstraction. Our latest Dezeen guide explains the seven key types of bridges including beam bridges, tied-arch bridges, cable-stayed bridges and cantilevered bridges. If there is a need to share an object among multiple other objects, then the Bridge pattern is a perfect fit for this job.
Design Patterns - Bridge Pattern
It is commonly used in industry as it is an easy way to simplify classes by separating the low-level logic implementation from the high-level definition of a class (which is usually abstracted/inherited). The purpose of this guide is to explain this pattern in three easy, clean steps. We have a DrawAPI interface which is acting as a bridge implementer and concrete classes RedCircle, GreenCircle implementing the DrawAPI interface. BridgePatternDemo, our demo class will use Shape class to draw different colored circle.
With this approach, your number of classes will increase over time. For example, including one more color would add two more shape classes. Bridge design pattern is most applicable in applications where we need to provide the components that are platform independence.
Recycled-aluminium objects
Doing so will make your code more flexible and less fragile to changes. Also, with the bridge pattern in place, it will be much easier to unit test your code. Without the bridge pattern, in order to test implementation classes, you need their super classes.

Key Milan design week installations
I’ll give you one new example for a bridge pattern if you get board of the same old Shape and Color example. Another advantage is that you can define Aimpl in a separate header file that need not be included by the users of A. All you have to do is use a forward declaration of Aimpl before A is defined, and move the definitions of all the member functions referencing pImpl into the .cpp file.
Terra AI "compass" enables users to take phone-free walks
Truss bridges feature a load-bearing superstructure made from steel elements interconnected in triangular arrangements to act as a single structural member. Tied-arch bridges are bridges that have arched structures that usually rise above the bridge's deck to support it from above via ties or hangers. These bridges work by using the arched form to transfer weight to abutments on either side of the structure.
Commuters enter day two of Washington Bridge traffic pattern change - WLNE-TV (ABC6)
Commuters enter day two of Washington Bridge traffic pattern change.
Posted: Wed, 10 Apr 2024 07:00:00 GMT [source]
The Bridge pattern is a composite of the Template and Strategy patterns. Here, we can notice that the View class has two methods show() and buttons() which are overridden in the Concrete Abstraction (Here, LongView class). Also, the View class has a reference (or bridge) to the Resource class.
For example, a basic remote control might only have two buttons, but you could extend it with additional features, such as an extra battery or a touchscreen. The base remote control class declares a reference field that links it with a device object. All remotes work with the devices via the general device interface, which lets the same remote support multiple device types. This example illustrates how the Bridge pattern can help divide the monolithic code of an app that manages devices and their remote controls.
Further, if you notice, the constructor takes one parameter of ILEDTV type, i.e., an instance of one of the child classes of ILEDTV type, which we want to access remotely. Further, we are passing that ILEDTV object to the base class constructor. Create a class file named SamsungRemoteControl.cs and copy and paste the following code.
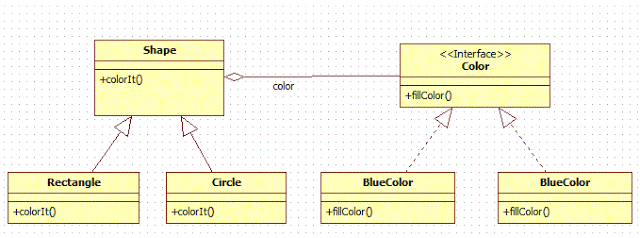
Weekly updates on the latest design and architecture vacancies advertised on Dezeen Jobs. Daily updates on the latest design and architecture vacancies advertised on Dezeen Jobs. ARCVS recently proposed a two-deck truss bridge for Novi Sad in Serbia that features offices and a hotel as well as a pedestrian crossing. Typically each span will either have a single arch with decks slung on each side or a double arch located on either side of the deck. Brearley Architects + Urbanists recently completed a beam bridge in China that incorporates spaces for play and resting.
Now, let's look at the implementation of the above-explained pseudocode in Java. Hence, we need a way to avoid this permanent binding between an abstraction and its implementation. When there is only one fixed implementation, this pattern is known as the Pimpl idiom in the C++ world. Use the Bridge pattern when you want to divide and organize a monolithic class that has several variants of some functionality (for example, if the class can work with various database servers). In a worst-case scenario, this app might look like a giant spaghetti bowl, where hundreds of conditionals connect different types of GUI with various APIs all over the code.
On the other hand, the abstraction takes an instance of the implementation class and runs its method. The Bridge pattern lets you split the monolithic class into several class hierarchies. After this, you can change the classes in each hierarchy independently of the classes in the others. This approach simplifies code maintenance and minimizes the risk of breaking existing code. The Bridge design pattern is a powerful tool to help you write more flexible and extensible code.
E.g. shapes and drawing methods, behaviours and platforms, file formats and serializers and so forth. Although it’s optional, the Bridge pattern lets you replace the implementation object inside the abstraction. Bridge supports may take the form of columns, towers or even the walls of a canyon. A cable-stayed bridge has one or multiple towers or pylons from which cables extend to support a bridge deck. The cables on these bridges often have a fan-like design or pattern caused by the cables forming parallel and regular lines as they extend to the deck.
This enables to configure an Abstraction with an Implementor object at run-time.See also the Unified Modeling Language class and sequence diagram below. Use the Bridge if you need to be able to switch implementations at runtime. Use the pattern when you need to extend a class in several orthogonal (independent) dimensions. The bigger a class becomes, the harder it is to figure out how it works, and the longer it takes to make a change. The changes made to one of the variations of functionality may require making changes across the whole class, which often results in making errors or not addressing some critical side effects.
No comments:
Post a Comment